Snapshot testing react components using Jest
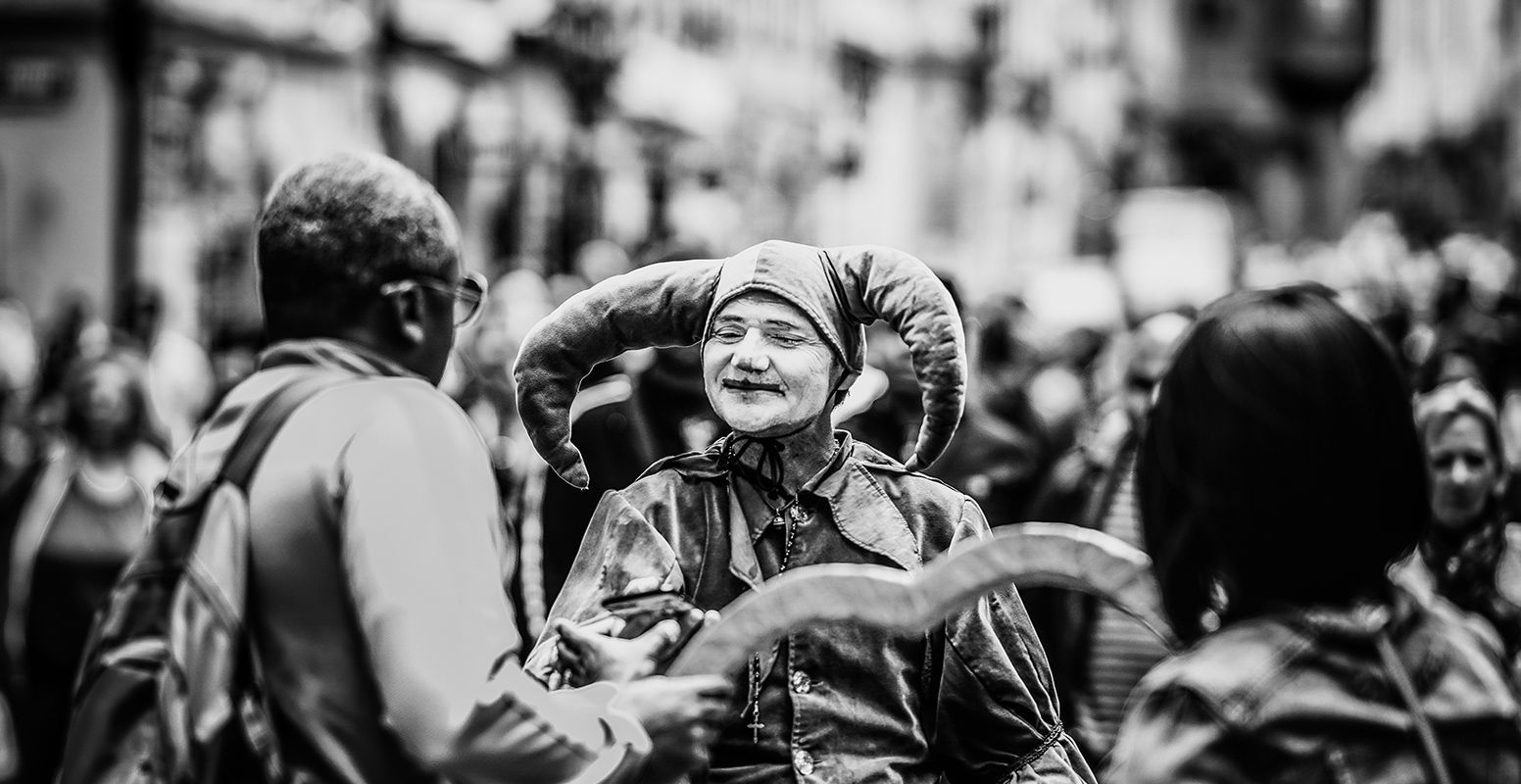
Testing a single page application can be quite hard when it comes to validating the HTML that is generated by your components. Snapshot testing can be quite helpful, especially in larger applications. Here's a short guide on how to use it in your React application!
What is snapshot testing and why should I use it?
Snapshot testing in React is a test method where we run a test and store a snapshot of the rendered HTML. Next time we run the test, we can verify that the HTML output of the component hasn't changed compared to the last time we ran the test.
The purpose of snapshot testing is to make sure you didn't break the layout of your component when you change behavior. Snapshot testing is great as an addon if you're already writing behavior driven tests.
Now that you understand what snapshot testing is, let's look at how you can implement it in Jest, a testing framework for React.
How does snapshot testing work?
In React, you can use Jest as a testing framework for writing unit-tests, integration tests and even end-to-end tests. Here's an example test written in Jest:
import React from 'react'; import App from './App';
describe(‘App’, () => { test(‘loads without error’, () => { let element = <App/>;
expect(element).toBeTruthy(); });
});
This test loads up React and the App component. It then runs a test case to check whether the App component loads as expected. Not very fancy, but great for demonstration purposes.
Let’s say you want to check whether the HTML generated by the app component changes when you change behavior. You can write the following additional test case for this:
import React from ‘react’; import renderer from ‘react-test-renderer’;
import App from ’./App’;
describe(‘App’, () => { test(‘render matches snapshot’, () => { const component = renderer.create(<App/>); const tree = component.toJSON();
expect(tree).toMatchSnapshot(); });
});
First, this test case creates a new test renderer for the App component. Next, it renders the component tree to JSON. Finally, it uses the toMatchSnapshot
matcher to verify the generated HTML against the stored snapshot.
The first time you run the test using the command jest
, a snapshot is stored on disk. Rendering the test green in the process, because there’s no snapshot to validate against.
The next time you run the test, the component is rendered again, but this time it is checked against a snapshot stored in the snapshots
folder.
When the generated tree matches the snapshot, the test is flagged green. If it doesn’t it will get flagged red.
It may very well happen that you’ve changed the HTML your component generates. That’s fine, run jest —updateSnapshot
to update the snapshot and commit the snapshots
folder to git so you’ll have an updated snapshot to work with the next time you run your tests.
Want to learn more?
That’s it for using snapshot tests in Jest. It’s a very powerful mechanism, especially if you’re building larger web applications in React or other single-page-application frameworks.
You can learn more about it in the Jest manual here: https://jestjs.io/docs/en/snapshot-testing